Summary: in this tutorial, you will learn how to open the file in Perl using the open()
function. We are going to show you how to open the file for reading and writing with error handling.
Perl filehandle
A filehandle is a variable that associates with a file. Through a filehandle variable, you can read from the file or write to the file depending on how you open the file.
Perl open file function
You use open()
function to open files. The open()
function has three arguments:
-
Filehandle
that associates with the file Mode
: you can open a file for reading, writing or appending.Filename
: the path to the file that is being opened.
open(filehandle,mode,filename)
Code language: Perl (perl)
To open a file in a specific mode, you need to pass the corresponding operand to the open()
function.
mode | operand |
---|---|
read | < |
write | > |
append | >> |
The open file modes are explained in details as follows:
- Read mode (<): you only can read the file but cannot change its content.
- Write mode (>): If the file does not exist, a new file is created. If the file already exists, the content of the file is wipe out, therefore, you should use the write mode with extra cautious.
- Append mode ( >>): as its name implied, you can open the file for appending new content to the existing content of the file. However, you cannot change the existing content in the file.
The following example demonstrates how to open the c:\temp\test.txt
file for reading using the open()
function.
open(FH, '<', 'c:\temp\test.txt');
Code language: Perl (perl)
The open file returns true
on success and false
on failure. You can use the die()
function to handle a file-opening failure. See the below example:
open(FH, '<', 'c:\temp\test.txt') or die $!;
Code language: Perl (perl)
$!
is a special variable that conveys the error message telling why the open()
function failed. It could be something like “No such file or directory” or “Permission denied”. In case the file c:\temp\test.txt
does not exist, you get an error message “No such file or directory”.
Closing the files
After processing the file such as reading or writing, you should always close it explicitly by using the close()
function. If you don’t, Perl will automatically close the file for you, however, it is not a good programming practice. The filehandle should always be closed explicitly.
Let’s a look at the following example:
#!/usr/bin/perl
use warnings;
use strict;
my $filename = 'c:\temp\test.txt';
open(FH, '<', $filename) or die $!;
print("File $filename opened successfully!\n");
close(FH);
Code language: Perl (perl)
If you have a file with name test.txt
resides in the folder c:\temp
, you will get the following output:
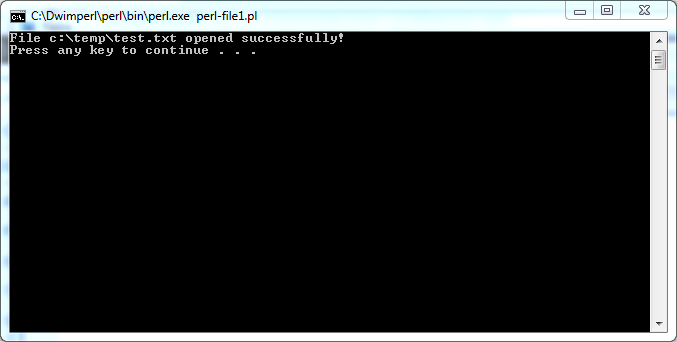
In this tutorial, you have learned how to open a file, close a file and handle error.